How Do You Know How Many Passes Through an Array It Will Take to Find a Value
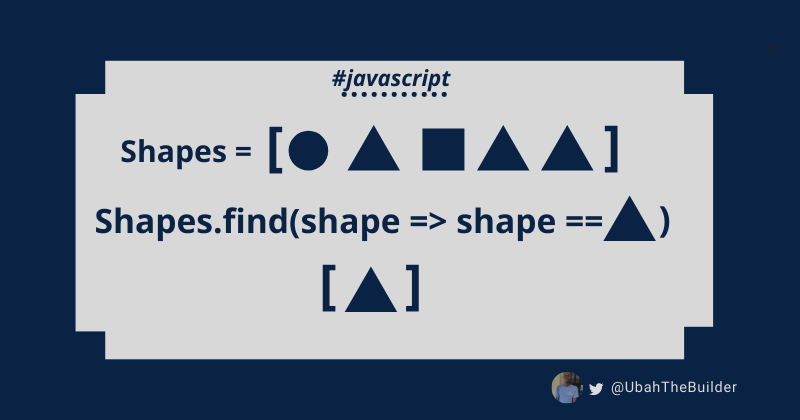
When you're working with an assortment collection, sometimes yous'll only need to find out if an item exists in the array so you can think information technology. And you won't care how many other items (if any) exist within the same assortment.
Well, we tin can use the find()
method to exercise just that.
How the Assortment.observe() Method Works
The detect()
method is an Array.epitome
(aka built-in) method which takes in a callback function and calls that role for every detail it iterates over inside of the assortment it is jump to.
When information technology finds a match (in other words, the callback function returns truthful
), the method returns that item array item and immediately breaks the loop. So the find()
method returns the showtime element inside an array which satisfies the callback function.
The callback function tin can take in the following parameters:
-
currentItem
: This is the element in the array which is currently beingness iterated over. -
index
: This is the index position of thecurrentItem
within the array. -
assortment
: This represents the target array forth with all its items.
How to Apply the observe()
Method in JavaScript
In the following examples, I will demonstrate how you can use the find()
method to retrieve the showtime particular from an assortment which matches a specified condition in JavaScript.
How to become a unmarried item with find()
Let'south assume yous take a dog which goes missing. You report it to the relevant government and they join a grouping of recovered dogs.
To be able to find your dog, you lot need to provide unique information about him. For example, the breed of your dog (a Chihuahua) might be used to identify information technology.
Nosotros tin limited this scenario in JavaScript using an array collection. The array called foundDogs
will incorporate all the names of the recovered dogs every bit well equally their respective breeds. And we'll utilise the detect()
method to find the domestic dog which is a Chihuahua from inside the assortment.
let foundDogs = [{ breed: "Beagle", color: "white" }, { breed: "Chihuahua", color: "yellow" }, { brood: "Pug", color: "black" }, ] office findMyDog(dog) { return canis familiaris.breed === "Chihuahua" } allow myDog = foundDogs.find(canis familiaris => findMyDog(dog)); panel.log(myDog); /* { breed: "Chihuahua", color: "yellowish" } */
The notice method stops iterating when a match is found.
There is something very important to remember almost discover()
: it stops executing one time the callback function returns a truthful
statement.
To illustrate this, we volition again employ the missing dog case. This fourth dimension, we will assume that two Chihuahuas were constitute.
Merely the find()
method will only return the first case of Chihuahua it discovers within the array. Whatsoever other instance will then be later on ignored.
Nosotros can also easily come across this past logging the index position of that item into the console:
let foundDogs = [{ breed: "Beagle", color: "white" }, { breed: "Chihuahua", color: "xanthous" }, { breed: "Pug", color: "blackness" }, { breed: "Chihuahua", color: "yellow" } ] function findMyDog(dog, index) { if (canis familiaris.breed === "Chihuahua") console.log(index); render dog.breed === "Chihuahua" } allow myDog = foundDogs.find((domestic dog, index) => findMyDog(dog, index)); console.log(myDog); /* 1 { breed: "Chihuahua", colour: "yellow" } */
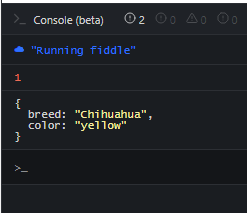
How to employ a Destructuring Assignment
Y'all can make your code more curtailed past combining both the destructuring assignment and an arrow function expression.
We'll employ destructuring to extract just the proper name property from the object which nosotros then pass in as a parameter to the callback function.
We'll get the aforementioned result:
let foundDogs = [{ breed: "Beagle", color: "white" }, { breed: "Chihuahua", color: "yellowish" }, { brood: "Pug", color: "blackness" }, ] let myDog = foundDogs.notice(({breed}) => breed === "Chihuahua"); console.log(myDog); /* { brood: "Chihuahua", colour: "yellow" } */
How to find an particular past its index
In this instance, we will exist finding and returning the spot belonging to 'David' from inside the assortment using its unique index value. This demonstrates one mode we tin apply the index
property inside of our callback
function with the find()
method:
allow reservedPositions = [{ name: "Anna", age: 24 }, { name: "Beth", age: 22 }, { proper noun: "Cara", age: 25 }, { proper noun: "David", age: xxx }, { name: "Ethan", historic period: 26 } ] function findByIndex(person, index) { return index === 3 } permit myPosition = reservedPositions.notice((person, index) => findByIndex(person, index)); console.log(myPosition); /* { historic period: xxx, proper noun: "David" } */
You Can Utilize the Context Object with find()
In addition to the callback function, the find()
method tin likewise take in a context object.
find(callback, contextObj)
We tin and so refer to this object from inside the callback part on each iteration, using the this
keyword as a reference. This allows us to admission any properties or methods defined inside of the context object.
How to use the context object with discover()
Allow's say nosotros take an assortment of job applications and want to select just the first applicant who meets all of the criteria.
All criteria is divers within a context object called criteria
and that object is later on passed as a second parameter into the find()
method. So, from inside the callback office, we access the object to check if an applicant matches all of the criteria specified there.
allow applicants = [ {proper noun: "aaron", yrsOfExperience: 18, historic period: 66}, {name: "beth", yrsOfExperience: 0, age: 18}, {proper name: "cara", yrsOfExperience: iv, age: 22}, {name: "daniel", yrsOfExperience: 3, age: sixteen}, {proper noun: "ella", yrsOfExperience: five, historic period: 25}, {name: "fin", yrsOfExperience: 0, historic period: 16}, {name: "george", yrsOfExperience: 6, historic period: 28}, ] allow criteria = { minimumExperience: 3, lowerAge: 18, upperAge: 65 } allow luckyApplicant = applicants.detect(function(bidder) { return applicant.yrsOfExperience >= this.minimumExperience && applicant.age <= this.upperAge && applicant.historic period >= this.lowerAge ; }, criteria) console.log(luckyApplicant); /* { age: 22, name: "cara", yrsOfExperience: four } */
Technically, iii applicants (Cara, Ella and George) all qualify based on the criteria. In other words, the three of them are at to the lowest degree xviii years old, not older than 65, and take at to the lowest degree three years of working feel.
Withal since the find()
method e'er returns ONLY the first instance which evaluates to true, the other ii will be ignored and the loop will exist broken.
Wrapping Upwardly
The find()
method is an Array.paradigm
method which takes in a callback role and calls that function for every detail inside the bound array.
When the callback role evaluates to true
, the method returns the current item and breaks the loop. It returns just the start match – whatsoever other matches present inside of the assortment will exist ignored.
In addition to the callback function, the find()
method tin also take in a context object as the second argument. This volition enable y'all admission any of its properties from the callback role using this
.
I hope y'all got something useful from this article.
I f you want to larn more about Web Evolution, feel gratis to visit my blog .
Thank you for reading and run into yous soon.
P/Southward : If y'all are learning JavaScript, I created an eBook which teaches 50 topics in JavaScript with hand-fatigued digital notes. Check it out here.
Learn to code for free. freeCodeCamp's open up source curriculum has helped more than than forty,000 people become jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/javascript-array-find-tutorial-how-to-iterate-through-elements-in-an-array/
Postar um comentário for "How Do You Know How Many Passes Through an Array It Will Take to Find a Value"